Connected Scatterplot
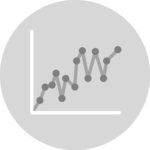
A connected scatterplot displays the evolution of a numeric variable. Data points are represented by a dot and connected with straight line segments. A variation of the connected scatterplot allows to study the evolution of 2 numeric variables together.
This page explains how to build a connected scatterplot using react
andd3.js
. It is highly connected with the line chart section of the gallery but provides further information concerning connected scatterplot specific features.
The Data
The dataset required to build a connected scatterplot is the same as for a line chart. It is usually an array where each item is an object providing the x
and the y
values of the data point.
Here is a minimal example:
const data = [
{x:1, y: 90},
{x: 2, y: 12},
{x: 3, y: 34},
{x: 4, y: 53},
{x: 5, y: 98},
]
Note: if your data is in .csv
format, you can translate it thanks to the d3.csv()
function as suggested here.
Note: a line chart is often made to represent time. If your x
property is a date, please visit the timeseries section.
Component skeleton
The goal here is to create a ConnectedScatterplot
component that will be stored in a ConnectedScatterplot.tsx
file. This component requires 3 props to render: a width
, a height
, and some data
.
The shape of the data
is described above. The width
and height
will be used to render an svg
element in the DOM, in which we will insert the Connected Scatterplot.
To put it in a nutshell, that's the skeleton of our ConnectedScatterplot
component:
import * as d3 from "d3"; // we will need d3.js
type ConnectedScatterplotProps = {
width: number;
height: number;
data: {x: number, y: number}[];
};
export const ConnectedScatterplot = ({ width, height, data }: ConnectedScatterplotProps) => {
// read the data
// build the scales and axes
// build the lines and circles
return (
<div>
<svg width={width} height={height}>
// render axes
// render all the <path>
</svg>
</div>
);
};
It's fundamental to understand that with this code organization, d3.js will be used to prepare the SVG path
, but it's React that will render them in the return()
statement. We won't use d3 methods like append
that you can find in usual d3.js examples.
Basic connected scatterplot
All the explanation about margins, scales, axes and d3.line()
are already made in the line chart section of the gallery.
The only thing we need to add here is some circles for each data point. The process is exactly the same as for a scatterplot. We have to loop through the data and a circle
for each item using the x and y scales:
Most basic line chart made with react (rendering) and d3.js (path computation)
Note: you can compare this with a d3.js only approach
Responsive Connected Scatter with react
The component above is not responsive. It expects 2 props called width
and height
and will render a Connected Scatter of those dimensions.
Making the Connected Scatter responsive requires adding a wrapper component that gets the dimension of the parent div
, and listening to a potential dimension change. This is possible thanks to a hook called useDimensions
that will do the job for us.
useDimensions
: a hook to make your viz responsive
export const useDimensions = (targetRef: React.RefObject<HTMLDivElement>) => {
const getDimensions = () => {
return {
width: targetRef.current ? targetRef.current.offsetWidth : 0,
height: targetRef.current ? targetRef.current.offsetHeight : 0
};
};
const [dimensions, setDimensions] = useState(getDimensions);
const handleResize = () => {
setDimensions(getDimensions());
};
useEffect(() => {
window.addEventListener("resize", handleResize);
return () => window.removeEventListener("resize", handleResize);
}, []);
useLayoutEffect(() => {
handleResize();
}, []);
return dimensions;
}
I'm in the process of writing a complete blog post on the topic. Subscribe to the project to know when it's ready.
ToDoadd links to line chart examples
ToDoreproduce the connected scatter from the state of JS survey
Connected Scatter inspiration
If you're looking for inspiration to create your next Connected Scatter, note that dataviz-inspiration.com showcases many examples. Definitely the best place to get ... inspiration!
dataviz-inspiration.com showcases hundreds of stunning dataviz projects. Have a look to get some ideas on how to make your Connected Scatter looks good!
visitContact
👋 Hey, I'm Yan and I'm currently working on this project!
Feedback is welcome ❤️. You can fill an issue on Github, drop me a message on Twitter, or even send me an email pasting yan.holtz.data
with gmail.com
. You can also subscribe to the newsletter to know when I publish more content!